Firebase CRUD Operations With Flutter Part 3 (Building Queries)
in this tutorial set we will see how to build Firestore Queries with flutter. we will also see how to create simple queries in Firestore. this will be the 3rd Part of Firebase CRUD Operations With Flutter. Read the Previous ones from Here.
Note : All the Working State Code for these tutorials can be found in : https://github.com/SrilalS/Flutter-Tutorial

Firestore is a NoSQL Database. but it doesn’t mean we can run Queries in the Database. We can run Many Types of Queries in the Firestore.
it Supports the following Queries.
isEqualTo : check if a value in the database is equal to a value we already have.
isLessThan : this works for numerical values.
isLessThanOrEqualTo : this works for numerical values.
isGreaterThan : this works for numerical values.
isGreaterThanOrEqualTo : this works for numerical values.
isNull : checks if value is null or not
Lets see how to build a simple query.
Firestore.instance.collection(‘TUT’).where(‘F’, isEqualTo: text).snapshots(),
i’m using the same database i used previously. here is the structure,

in the Query i made, it checks the field ‘F’ in the Documents in the TUT Collection against the local variable “text”.
here is the full example.
here it only returns the ‘UNN’ ships. isEqual works for many Datatypes unlike other Queries.

lets see a example of isGreaterThan.
Firestore.instance.collection(‘TUT’).where(‘C’,isGreaterThan: amount).snapshots(),
the variable “amount” has the value of 1500, so It returns all the ships with crew capacity more then 1500,
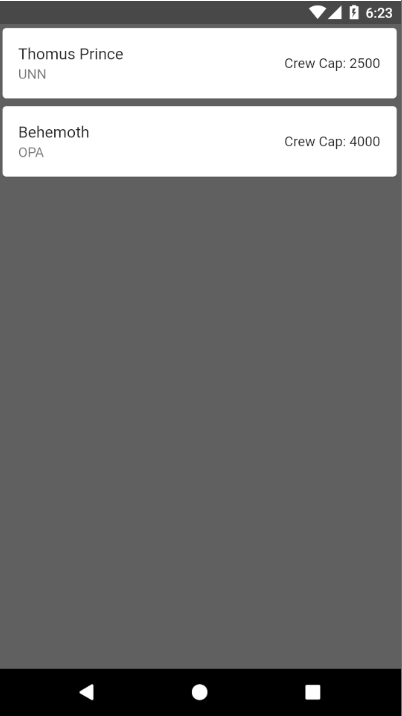
You can also combine multiple queries like below.
Firestore.instance.collection(‘TUT’).where(‘F’,isEqualTo: text).where(‘C’,isGreaterThan: amount).snapshots(),